[문제]
https://www.acmicpc.net/problem/16173
16173번: 점프왕 쩰리 (Small)
쩰리는 맨 왼쪽 위의 칸에서 출발해 (행, 열)로 나타낸 좌표계로, (1, 1) -> (2, 1) -> (3, 1) -> (3, 3)으로 이동해 게임에서 승리할 수 있다.
www.acmicpc.net
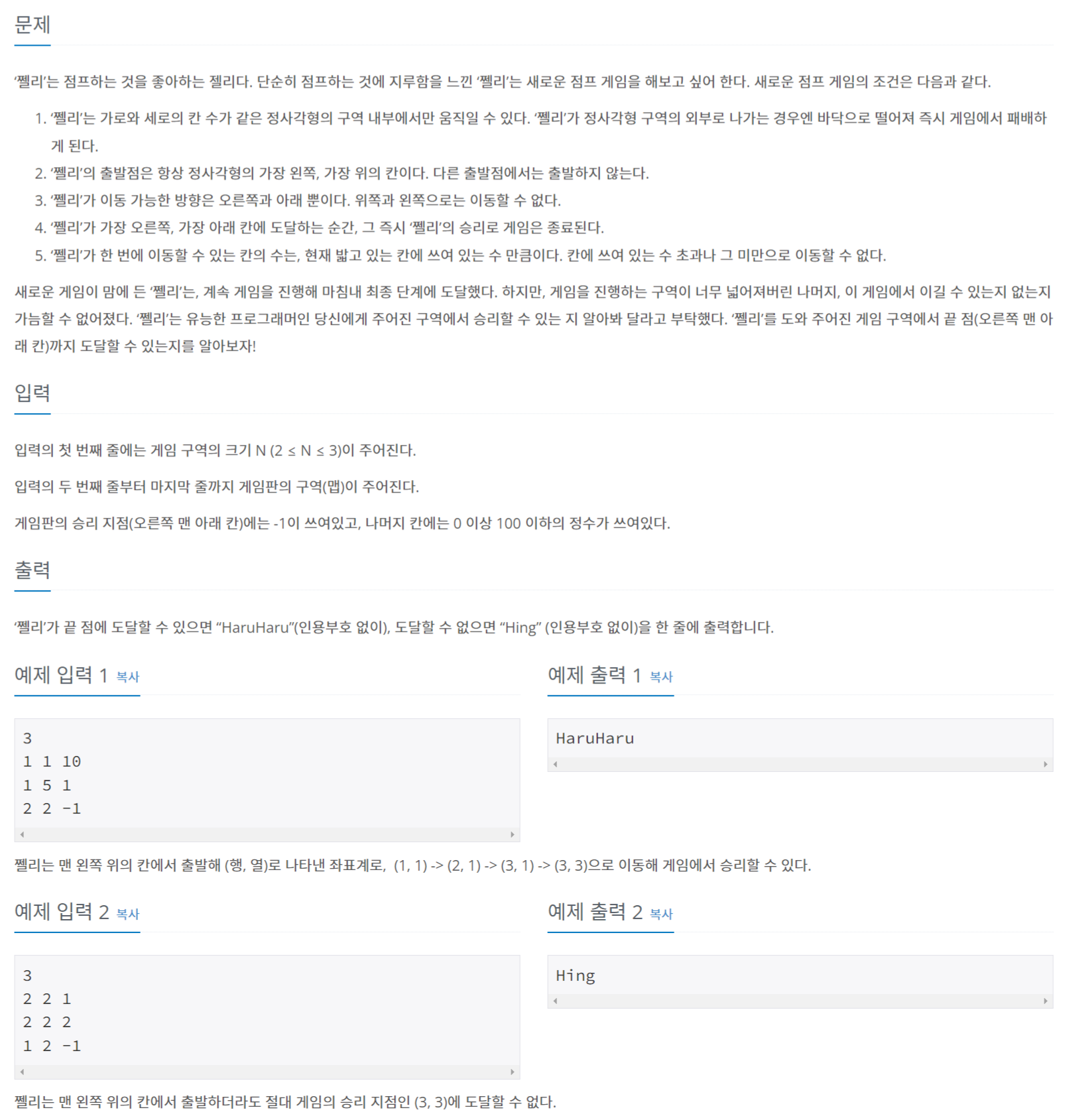
[풀이]
간단한 dfs 문제였는데 exit(0)을 생각을 못해서 좀 걸렸다
우선 이렇게 풀어놓았지만 exit 을 이용하지 않고 제대로 푸는 방법을 생각해봐야겠다 -> 수정 완료
[코드]
import sys
n = int(sys.stdin.readline())
square = []
for _ in range(n):
square.append(list(map(int, sys.stdin.readline().split())))
visited = [[False] * n for _ in range(n)]
def dfs(x, y):
if x >= n or y >= n:
return False
value = square[x][y]
if value == -1:
print("HaruHaru")
exit(0)
if not visited[x][y]:
visited[x][y] = True
dfs(x + value, y) # 아래
dfs(x, y + value) # 오른쪽
dfs(0, 0)
print("Hing")
[개선된 코드]
import sys
from collections import deque
def solution(N, graph):
visited = [[False] * N for _ in range(N)]
dx = [1, 0]
dy = [0, 1]
def dfs(x, y, visited):
queue = deque()
queue.append((x, y))
while queue:
x, y = queue.popleft()
if graph[x][y] == -1:
return 'HaruHaru'
jump = graph[x][y] # 점프 값
for i in range(2):
nx = x + dx[i] * jump
ny = y + dy[i] * jump
if nx >= N or ny >= N:
continue
if visited[nx][ny]:
continue
else:
visited[nx][ny] = True
queue.append((nx, ny))
return 'Hing'
print(dfs(0, 0, visited))
if __name__ == "__main__":
N = int(sys.stdin.readline())
graph = [list(map(int, sys.stdin.readline().split())) for _ in range(N)]
solution(N, graph)
'문제풀이 > BOJ' 카테고리의 다른 글
[Python] BOJ/백준 7662번 이중 우선순위 큐 (0) | 2022.06.24 |
---|---|
[Python] BOJ/백준 7576번 토마토 (0) | 2022.06.22 |
[Python] BOJ/백준 1003번 피보나치 함수 (0) | 2022.03.04 |
[Python] BOJ/백준 1620번 나는야 포켓몬 마스터 이다솜 (0) | 2022.03.04 |
[Python] BOJ/백준 11659번 구간 합 구하기 4 (0) | 2021.09.04 |
[문제]
https://www.acmicpc.net/problem/16173
16173번: 점프왕 쩰리 (Small)
쩰리는 맨 왼쪽 위의 칸에서 출발해 (행, 열)로 나타낸 좌표계로, (1, 1) -> (2, 1) -> (3, 1) -> (3, 3)으로 이동해 게임에서 승리할 수 있다.
www.acmicpc.net
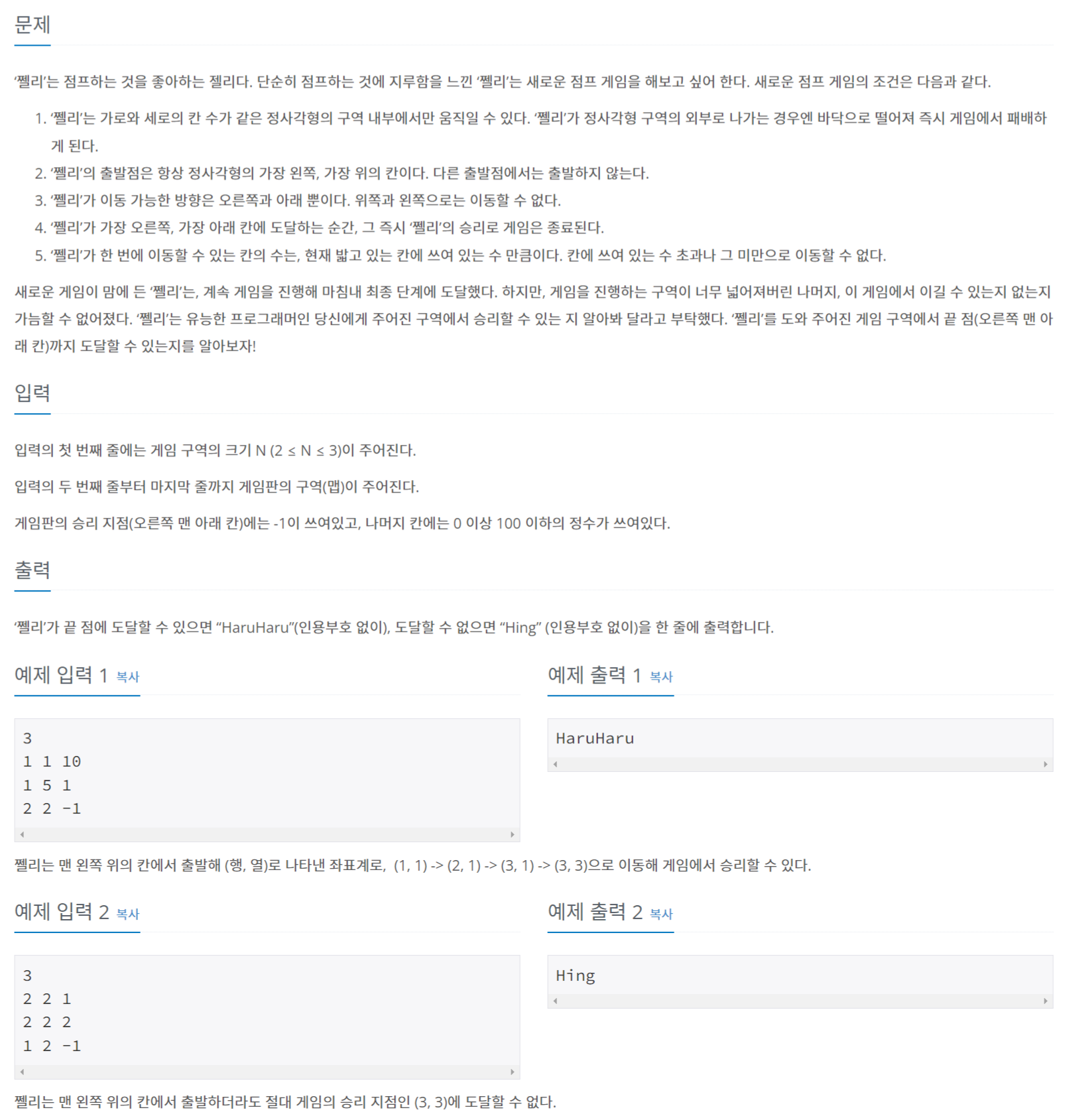
[풀이]
간단한 dfs 문제였는데 exit(0)을 생각을 못해서 좀 걸렸다
우선 이렇게 풀어놓았지만 exit 을 이용하지 않고 제대로 푸는 방법을 생각해봐야겠다 -> 수정 완료
[코드]
import sys
n = int(sys.stdin.readline())
square = []
for _ in range(n):
square.append(list(map(int, sys.stdin.readline().split())))
visited = [[False] * n for _ in range(n)]
def dfs(x, y):
if x >= n or y >= n:
return False
value = square[x][y]
if value == -1:
print("HaruHaru")
exit(0)
if not visited[x][y]:
visited[x][y] = True
dfs(x + value, y) # 아래
dfs(x, y + value) # 오른쪽
dfs(0, 0)
print("Hing")
[개선된 코드]
import sys
from collections import deque
def solution(N, graph):
visited = [[False] * N for _ in range(N)]
dx = [1, 0]
dy = [0, 1]
def dfs(x, y, visited):
queue = deque()
queue.append((x, y))
while queue:
x, y = queue.popleft()
if graph[x][y] == -1:
return 'HaruHaru'
jump = graph[x][y] # 점프 값
for i in range(2):
nx = x + dx[i] * jump
ny = y + dy[i] * jump
if nx >= N or ny >= N:
continue
if visited[nx][ny]:
continue
else:
visited[nx][ny] = True
queue.append((nx, ny))
return 'Hing'
print(dfs(0, 0, visited))
if __name__ == "__main__":
N = int(sys.stdin.readline())
graph = [list(map(int, sys.stdin.readline().split())) for _ in range(N)]
solution(N, graph)
'문제풀이 > BOJ' 카테고리의 다른 글
[Python] BOJ/백준 7662번 이중 우선순위 큐 (0) | 2022.06.24 |
---|---|
[Python] BOJ/백준 7576번 토마토 (0) | 2022.06.22 |
[Python] BOJ/백준 1003번 피보나치 함수 (0) | 2022.03.04 |
[Python] BOJ/백준 1620번 나는야 포켓몬 마스터 이다솜 (0) | 2022.03.04 |
[Python] BOJ/백준 11659번 구간 합 구하기 4 (0) | 2021.09.04 |